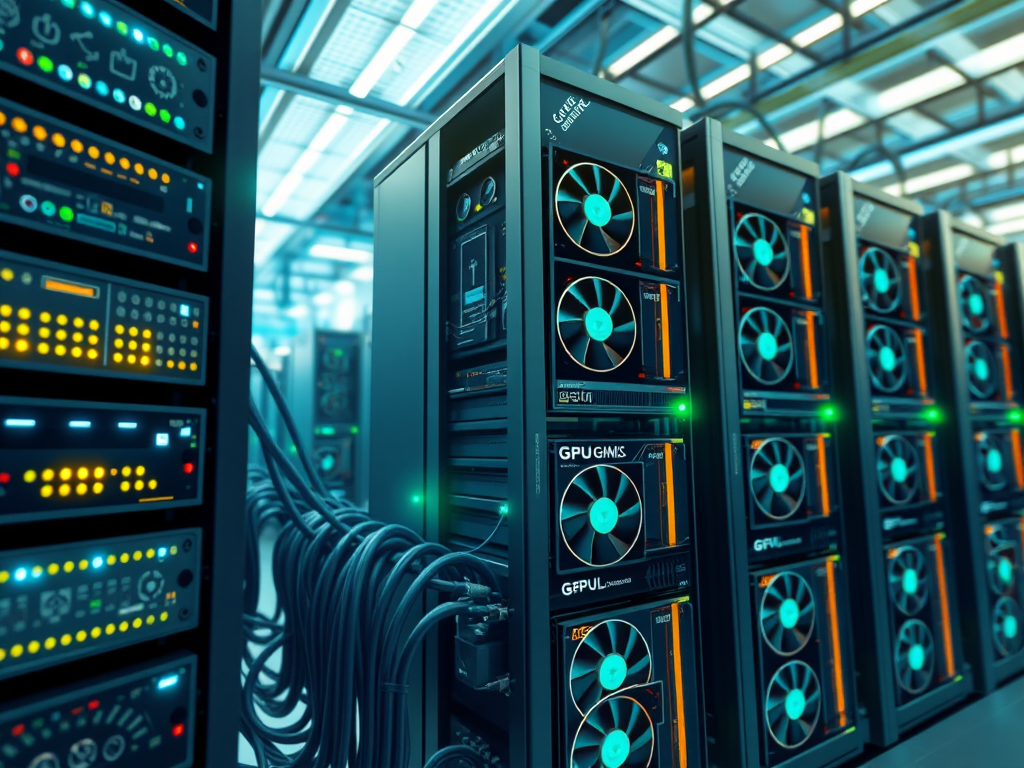
Deep learning is transforming many fields, such as computer vision, natural language processing, and robotics. However, to fully harness the power of deep learning frameworks on GPU clusters, you need to optimize how they run. This guide provides a detailed look at effective strategies for optimizing these frameworks, making it easier to achieve faster training times and better model performance.
1. Understanding GPU Architecture
1.1 What is CUDA and cuDNN?
NVIDIA’s CUDA (Compute Unified Device Architecture) is a platform that allows developers to use GPUs for general-purpose computing. It enables parallel processing, which is essential for deep learning tasks. Frameworks like TensorFlow and PyTorch rely on cuDNN, a GPU-accelerated library specifically designed for deep neural networks. By leveraging these tools, you can significantly speed up calculations involved in training models.
1.2 GPU Memory Management
GPUs have limited memory (often a few gigabytes), so managing this resource effectively is crucial. Techniques such as memory pooling help reduce fragmentation and ensure that memory is used efficiently. Additionally, keeping data close to where it will be processed minimizes the time spent transferring data between CPU and GPU.
2. Distributed Training
2.1 Data Parallelism
Data parallelism is a common approach where a large dataset is divided among multiple GPUs. Each GPU processes its subset of data and computes gradients independently. After that, the gradients are averaged to update the model parameters. This method is supported by most frameworks and is relatively straightforward to implement.
2.2 Model Parallelism
For very large models that don’t fit into a single GPU’s memory, model parallelism is necessary. This technique splits the model itself across several GPUs, allowing different parts of the model to be processed simultaneously. While more complex to set up, it is essential for large-scale deep learning applications.
2.3 Hybrid Approaches
Combining data and model parallelism can yield significant performance improvements. In this hybrid approach, you can split both the dataset and model, scaling your training process across many GPUs. This allows for efficient utilization of resources and can lead to faster training times.
3. Efficient Data Loading
3.1 Preprocessing and Augmentation
Data preprocessing, such as normalization and augmentation, should ideally be handled on the CPU. This offloads work from the GPU, allowing it to focus on model training. Techniques like multi-threading or asynchronous data loading can ensure that data is prepared in advance, keeping the GPU busy.
3.2 Use of TFRecord and Datasets
In TensorFlow, using TFRecord format can streamline data storage and retrieval, making it faster to load large datasets. For PyTorch users, leveraging torch.utils.data.Dataset
and DataLoader
can help manage data efficiently, ensuring that batches are ready when the model is ready to process them.
4. Hyperparameter Tuning
4.1 Automated Tuning
Finding the best hyperparameters can be time-consuming. Automated tuning tools like Optuna or Ray Tune can help search through the hyperparameter space efficiently. These tools can run multiple experiments in parallel, utilizing the GPU clusters effectively to speed up the process.
4.2 Learning Rate Scheduling
Adjusting the learning rate during training can greatly affect model performance. Learning rate schedulers help modify the learning rate based on training progress, helping to avoid overshooting minima and speeding up convergence. Implementing these can lead to better results in less time.
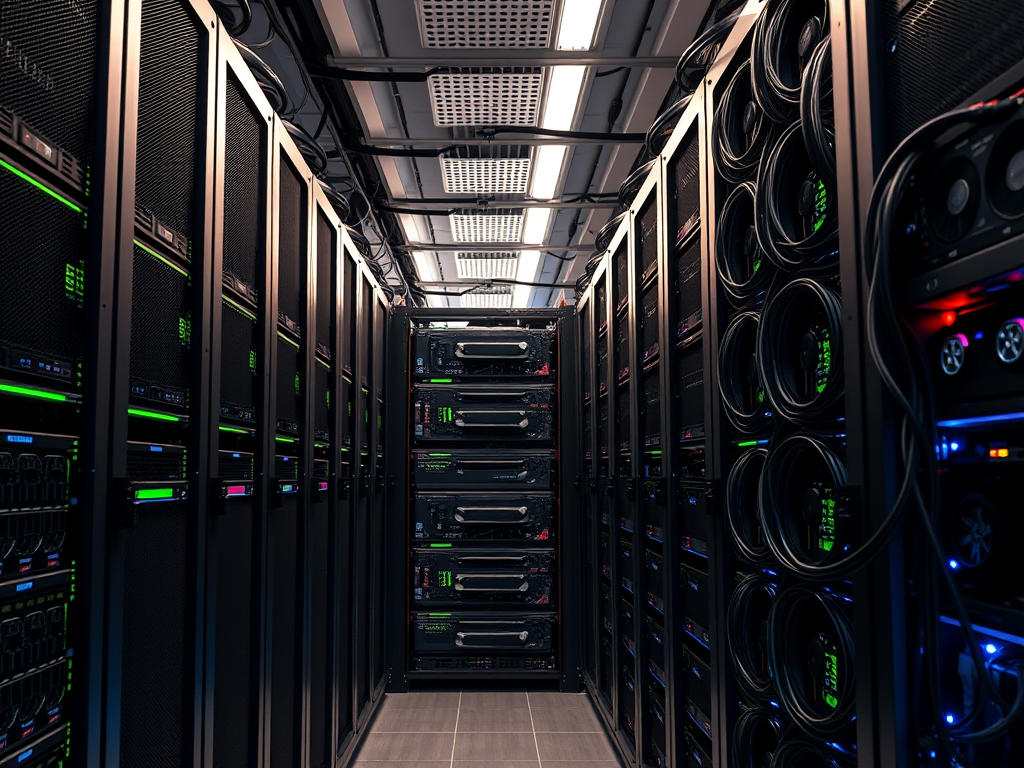
5. Profiling and Debugging
5.1 Tools and Libraries
Profiling tools like NVIDIA’s Nsight Systems and TensorBoard are invaluable for monitoring GPU utilization, memory usage, and identifying bottlenecks in your training process. These tools provide insights into how efficiently your resources are being used and where improvements can be made.
5.2 Benchmarking
Regular benchmarking of different configurations is crucial for understanding the impact of optimizations. By measuring training time, accuracy, and resource utilization, you can determine which setups yield the best performance. This iterative approach helps refine your training process over time.
6. Framework-Specific Optimizations
6.1 TensorFlow Optimizations
- Using
tf.function
: This feature allows you to compile parts of your model for better performance, reducing overhead during training. - Mixed Precision Training: Utilizing
tf.keras.mixed_precision
helps speed up computations by using lower precision (like float16) where possible, leading to faster training and reduced memory usage.
6.2 PyTorch Optimizations
- Using
torch.jit
: This just-in-time compilation feature can optimize your models for faster execution, making your training more efficient. - Distributed Training with
torch.distributed
: This module allows you to implement distributed training easily across multiple GPUs, enabling efficient scaling of your training jobs.
7. Advanced Techniques
7.1 Gradient Accumulation
For situations where batch sizes are limited by GPU memory, gradient accumulation allows you to simulate larger batch sizes. By accumulating gradients over several smaller batches before updating model weights, you can achieve the benefits of larger batch training without exceeding memory limits.
7.2 Early Stopping and Checkpointing
Implementing early stopping can save time by halting training when the model stops improving. Additionally, checkpointing allows you to save model states at regular intervals, so you can resume training later without losing progress.
7.3 Using Pre-trained Models
Leveraging pre-trained models can save a significant amount of time and computational resources. Fine-tuning these models for your specific task can lead to faster convergence and better performance, particularly when data is limited.
Conclusion
Optimizing deep learning frameworks for GPU clusters is a multifaceted task that involves understanding GPU architecture, managing data efficiently, and leveraging distributed training techniques. By tuning hyperparameters, using the right tools for profiling, and applying framework-specific optimizations, you can significantly enhance the performance of your deep learning models. Staying updated on the latest optimization strategies will help you maximize the potential of your GPU clusters, ultimately leading to faster and more accurate model training.